Snail-Kotlin alternatives and similar libraries
Based on the "Functional Programming" category.
Alternatively, view Snail-Kotlin alternatives based on common mentions on social networks and blogs.
-
Result
The modelling for success/failure of operations in Kotlin and KMM (Kotlin Multiplatform Mobile)
InfluxDB - Power Real-Time Data Analytics at Scale
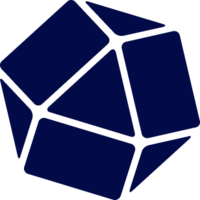
Do you think we are missing an alternative of Snail-Kotlin or a related project?
README
Snail-Kotlin 🐌

A lightweight observables framework, also available in Swift
Download
You can download a jar from GitHub's releases page.
Jitpack
allprojects {
repositories {
...
maven { url "https://jitpack.io" }
}
}
dependencies {
compile 'com.github.urbancompass:snail-kotlin:x.x.x'
}
Creating Observables
val observable = Observable<thing>()
Subscribing to Observables
observable.subscribe(
next = { thing in ... }, // do something with thing
error = { error in ... }, // do something with error
done = { ... } // do something when it's done
)
Closures are optional too...
observable.subscribe(
next = { thing in ... } // do something with thing
)
observable.subscribe(
error = { error in ... } // do something with error
)
Creating Observables Variables
val variable = Variable<whatever>(some initial value)
val optionalString = Variable<String?>(null)
optionalString.asObservable().subscribe(
next = { string in ... } // do something with value changes
)
optionalString.value = "something"
val int = Variable<Int>(12)
int.asObservable().subscribe(
next = { int in ... } // do something with value changes
)
int.value = 42
Miscellaneous Observables
val just = Just(1) // always returns the initial value (1 in this case)
val failure = Fail(RunTimeException()) // always returns error
failure.subscribe(
error = { it } //it is RuntimeException
)
val n = 5
let replay = Replay(n) // replays the last N events when a new observer subscribes
Dispatchers
You can specify which dispatcher an observables will be notified on by using .subscribe(dispatcher: <desired dispatcher>)
. If you don't specify, then the observable will be notified on the same dispatcher that the observable published on.
There are 3 scenarios:
You don't specify the dispatcher. Your observer will be notified on the same dispatcher as the observable published on.
You specified
Main
dispatcher AND the observable published on theMain
dispatcher. Your observer will be notified synchronously on theMain
dispatcher.You specified a dispatcher. Your observer will be notified async on the specified dispatcher.
Examples
Subscribing on Main
observable.subscribe(Dispatchers.Main, next = {
// do stuff with it...
})