PultusORM alternatives and similar libraries
Based on the "Database" category.
Alternatively, view PultusORM alternatives based on common mentions on social networks and blogs.
-
mapdb
MapDB provides concurrent Maps, Sets and Queues backed by disk storage or off-heap-memory. It is a fast and easy to use embedded Java database engine. -
DBFlow
A blazing fast, powerful, and very simple ORM android database library that writes database code for you. -
kotlin-gremlin-ogm
DISCONTINUED. Kotlin-gremlin-ogm is a type-safe object/graph mapping library for Gremlin enabled graph databases. -
kotlin-jpa-specification-dsl
This library provides a fluent DSL for querying spring data JPA repositories using spring data Specifications (i.e. the JPA Criteria API), without boilerplate code or a generated metamodel. -
zeko-sql-builder
Zeko SQL Builder is a high-performance lightweight SQL query library written for Kotlin language -
fluid-mongo
Kotlin coroutine support for MongoDB built on top of the official Reactive Streams Java Driver -
jds
Jenesis Data Store: a dynamic, cross platform, high performance, ORM data-mapper. Designed to assist in rapid development and data mining -
potassium-nitrite
Potassium Nitrite is a kotlin extension of nitrite database, an open source nosql embedded document store with mongodb like api.
InfluxDB - Power Real-Time Data Analytics at Scale
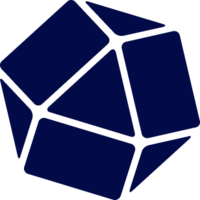
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of PultusORM or a related project?
README
BelleORM 
A Sqlite ORM library for Kotlin, Java & Android.
Status : Active Version : v1.8
Features
Currently implemented:
- Insert
- Retrieve
- Update
- Delete
- Drop
Usages
In your build file add
Gradle
allprojects {
repositories {
...
maven { url "https://jitpack.io" }
}
}
And
dependencies {
implementation 'ninja.sakib:BelleORM:v1.8'
}
Maven
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
And
<dependency>
<groupId>ninja.sakib</groupId>
<artifactId>BelleORM</artifactId>
<version>v1.8</version>
</dependency>
In case you need jar download is available here .
More option can be found here.
Examples
Open database connection
Kotlin
In Kotlin,
val belleORM: BelleORM = BelleORM("test.db", "/Users/s4kib/")
val belleORM: BelleORM = BelleORM("test.db") // DB will take place in user.home directory
In Android,
val appPath: String = getApplicationContext().getFilesDir().getAbsolutePath() // Output : /data/data/application_package_name/files/
val belleORM: BelleORM = BelleORM("test.db", appPath)
Java
In Java,
BelleORM belleORM = new BelleORM("test.db", "/Users/s4kib/")
BelleORM belleORM = new BelleORM("test.db", ) // DB will take place in user.home directory
In Android,
String appPath = getApplicationContext().getFilesDir().getAbsolutePath() // Output : /data/data/application_package_name/files/
val belleORM = new BelleORM("test.db", appPath)
Insert value
class Student {
@PrimaryKey
@AutoIncrement
var studentId: Int = 0
var name: String? = null
var department: String? = null
var cgpa: Double = 0.0
var dateOfBirth: java.util.Date? = null
@Ignore
var section: String? = null
}
val student: Student = Student()
student.name = "Sakib Sayem"
student.department = "CSE"
student.cgpa = 2.3
student.dateOfBirth = Date()
belleORM.save(student)
belleORM.close()
Retrieve Values
val students = belleORM.find(Student())
for (it in students) {
val student = it as Student
println(student.studentId)
println(student.name)
println(student.department)
println(student.cgpa)
println(student.dateOfBirth)
println()
}
Result
1
Sakib Sayem
CSE
2.3
Wed Sep 27 23:21:52 BDT 2017
Retrieve values based on condition
val condition: BelleORMCondition = BelleORMCondition.Builder()
.eq("name", "sakib")
.and()
.greaterEq("cgpa", 18)
.or()
.startsWith("name", "sami")
.sort("name", BelleORMQuery.Sort.DESCENDING)
.sort("department", BelleORMQuery.Sort.ASCENDING)
.build()
val students = belleORM.find(Student(), condition)
for (it in students) {
val student = it as Student
println("${student.studentId}")
println("${student.name}")
}
Update value
// values will be updated based on this condition
val condition: BelleORMCondition = BelleORMCondition.Builder()
.eq("name", "Sakib")
.build()
val updater: BelleORMUpdater = BelleORMUpdater.Builder()
.set("name", "Sayan Nur")
.condition(condition) // condition is optional
.build()
belleORM.update(Student(), updater)
Delete values
belleORM.delete(Student())
Drop Table
belleORM.drop(Student())
Check out more examples & API docs here
Note
Tables will be created on fly if not exists using class name and columns based on class fields. Currently supported types:
- String
- Int
- Long
- Float
- Double
- Boolean
- Date (java.util)
Autoincrement annotated fields values will be skipped as that will be handled by sqlite.
License
Copyright © Sakib Sami
Distributed under MIT license
Patreon Me !!!
If you want to support this project Patreon Me!
Buy Me a Coffee
*Note that all licence references and agreements mentioned in the PultusORM README section above
are relevant to that project's source code only.