kds alternatives and similar libraries
Based on the "Misc" category.
Alternatively, view kds alternatives based on common mentions on social networks and blogs.
-
jclasslib
jclasslib bytecode editor is a tool that visualizes all aspects of compiled Java class files and the contained bytecode. -
kotlin-logging
Lightweight Multiplatform logging framework for Kotlin. A convenient and performant logging facade. -
lingua
The most accurate natural language detection library for Java and the JVM, suitable for long and short text alike -
Kotlift
DISCONTINUED. Kotlift is the first source-to-source language transpiler from Kotlin to Swift -
Humanizer.jvm
Humanizer.jvm meets all your jvm needs for manipulating and displaying strings, enums, dates, times, timespans, numbers and quantities. -
klutter
A mix of random small libraries for Kotlin, the smallest reside here until big enough for their own repository. -
kassava
This library provides some useful kotlin extension functions for implementing toString(), hashCode() and equals() without all of the boilerplate. -
solr-undertow
Solr / SolrCloud running in high performance server - tiny, fast startup, simple to configure, easy deployment without an application server. -
SimpleDNN
SimpleDNN is a machine learning lightweight open-source library written in Kotlin designed to support relevant neural network architectures in natural language processing tasks -
kotlin-futures
A collections of extension functions to make the JVM Future, CompletableFuture, ListenableFuture API more functional and Kotlin like. -
kasechange
๐ซ๐๐ข๐ ฟ Multiplatform Kotlin library to convert strings between various case formats including Camel Case, Snake Case, Pascal Case and Kebab Case -
PrimeCalendar
PrimeCalendar provides all of the java.util.Calendar functionalities for Persian, Hijri, and ... dates. It is also possible to convert dates to each other.
WorkOS - The modern identity platform for B2B SaaS
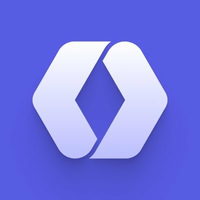
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of kds or a related project?
README
Kds is a Data Structure library for Multiplatform Kotlin 1.3. It includes a set of optimized data structures written in Kotlin Common so they are available in JVM, JS and future multiplatform targets. Those structures are designed to be allocation-efficient and fast, so Kds include specialized versions for primitives like Int or Double.
<!-- BADGES --> <!-- /BADGES -->
<!-- SUPPORT --> Support kds If you like kds, or want your company logo here, please consider becoming a sponsor โ , in addition to ensure the continuity of the project, you will get exclusive content. <!-- /SUPPORT -->
Full Documentation: https://korlibs.soywiz.com/kds/
Some samples:
// Case Insensitive Map
val map = mapOf("hELLo" to 1, "World" to 2).toCaseInsensitiveMap()
println(map["hello"])
// BitSet
val array = BitSet(100) // Stores 100 bits
array[99] = true
// TypedArrayList
val v20 = intArrayListOf(10, 20).getCyclic(-1)
// Deque
val deque = IntDeque().apply {
addFirst(n)
removeFirst()
addLast(n)
}
// CacheMap
val cache = CacheMap<String, Int>(maxSize = 2).apply {
this["a"] = 1
this["b"] = 2
this["c"] = 3
assertEquals("{b=2, c=3}", this.toString())
}
// IntIntMap
val m = IntIntMap().apply {
this[0] = 98
}
// Pool
val pool = Pool { Demo() }
pool.alloc { demo ->
println("Temporarilly allocated $demo")
}
// Priority Queue
val pq = IntPriorityQueue()
pq.add(10)
pq.add(5)
pq.add(15)
assertEquals(5, pq.removeHead())
// Extra Properties
class Demo : Extra by Extra.Mixin() { val default = 9 }
var Demo.demo by Extra.Property { 0 }
var Demo.demo2 by Extra.PropertyThis<Demo, Int> { default }
val demo = Demo()
assertEquals(0, demo.demo)
assertEquals(9, demo.demo2)
demo.demo = 7
assertEquals(7, demo.demo)
assertEquals("{demo=7, demo2=9}", demo.extra.toString())
// mapWhile
val iterator = listOf(1, 2, 3).iterator()
assertEquals(listOf(1, 2, 3), mapWhile({ iterator.hasNext() }) { iterator.next()})
// And much more!
Usage with gradle:
def kdsVersion = "..." //the latest version here (you can find it at the top of the README)
repositories {
maven { url "https://dl.bintray.com/korlibs/korlibs" }
}
dependencies {
// For multiplatform projects
implementation "com.soywiz.korlibs.kds:kds:$kdsVersion"
// For JVM/Android only
implementation "com.soywiz.korlibs.kds:kds-jvm:$kdsVersion"
// For JS only
implementation "com.soywiz.korlibs.kds:kds-js:$kdsVersion"
}
// settigs.gradle
enableFeaturePreview('GRADLE_METADATA')